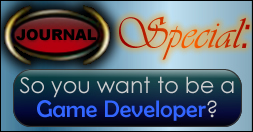
|
|
Entry #7: Execution is Everything |
|
July 13th, 2008 | Neil Rodrigues |
|
In last month’s entry, we discussed the software design of the dialogue system. This month’s entry continues with the programming theme, but we dive a bit deeper into the inner workings of the game. The dialogue system is a software component that interacts with the game engine, however it can still be thought of as a game element no different than backgrounds or animations. The game engine is like a container holding all the assets we’ve previously talked about. However, something still has to make sense of it all.
Something has to control what the player sees and hears at all times. Something has to monitor the actions of the player, and convert them into sequences or gameplay. Something has to continuously track everything the player does and tries to do, in order to asses how close or far he/she is from completing the game. If you haven’t already guessed it, this thing is scripting.
A scene’s script is not too different from the written script (a.k.a. the plot). It is slightly more structured and not quite as readable as written English. But, it’s not hard to understand once you get the hang of it. This month’s entry will show how we’ve scripted some scenes and sequences in The Silver Lining. |
|
|
Reference Material
The ultimate reference for scripting comes from none other than the written script. As previously mentioned, the syntax is very close to ordinary English, and with the exception of programming shorthand for comparisons, repetitions, and the return value of computations, it’s almost like reading a story.
The script is organized by sections of character interactions, based on the game cursor. For example: “LOOK at bed” refers to clicking the Eye cursor on the object in the scene that represents a bed. “HAND on cloak” refers to clicking the Hand cursor on the cloak. TALK, USE and WALK follow the same structure. |
|
|
Cursor Actions
Typically, Look interactions are Narrator dialogues, Hand interactions involve picking up objects, Walk interactions trigger scene transitions, Use interactions trigger sequences by using an object on something else, and Talk interactions represent communicating with other characters.
There are, of course, exceptions where a cursor can perform some other action, or combination of actions, depending on the scene and/or other conditions that may or may not have been met yet. This whole idea of interactions being both general and specific while remaining consistent throughout its usage is what gives scripting its “magic”.
The way the engine works is that it first checks for a specific interaction (scene-specific, that is). If one exists, it performs that and finishes. Otherwise, it falls back to the typical interaction, which is to check for dialogue specific to that object. If that fails, it will play the Narrator error message, basically meaning the action you were trying to perform was deemed nonsensical. To illustrate the relationship between the written script and a scene script, here is the interaction for clicking the Eye on Bookshop in Chapter 1:
Written script:
LOOK at bookshop:
NARRATOR: Ali's bookstore is located on the basement of a two-story building. Judging by what Graham can see through the window, the bookstore is well-stocked for such a small village. There is a note tacked to the door.
Game script:

Figure 1: Code for Isle of the Crown - VillageStreetStorm -
Look at Door to Bookshop
Since this interaction is the same as the general behavior, a scene-specific one is not required. One was used here, only because the object name was “DoorBookShop”, while the one entered into the Conversation Editor was named “Bookshop”. This mismatch sometimes occurs because the person building the set is different from the one inputting dialogue. It could have been corrected by changing the name in the conversation file to DoorBookShop, changing the object’s name to BookShop, or the third option of creating a handling function to equate them, as was the case here. The PlayTopic() method plays the same dialogue from the written script shown above. |
|
|
Sequences
The true value of scripting comes into play when implementing sequences. A sequence is essentially a series of timed events that occur after a cursor action. They are almost always scene-specific, since they involve objects and/or characters that only appear within a particular scene. Sequences are not reserved only for cut-scenes, as they can be used for anything that requires timing.
In Chapter 1, Hand on Bookshop triggers a seemingly simple sequence:
Written script:
HAND on bookshop door:
Sequence trigger:
EXT – VILLAGE STREET - DAY
Graham knocks on it
NARRATOR: No one answers.
(End of Sequence)
Game script:

Figure 2: Code for Isle of the Crown - VillageStreetStorm -
Hand on Door to Bookshop
As you can see, the scripting for this sequence contains a lot more than the written script. However, they are both essentially the same. For those of you unfamiliar with reading code, the scripted sequence consists of:
- making Graham walk to the Bookshop Door
- playing “knock on door” animation
- playing “knock on door” sound effect
- waiting for someone to respond to knocking
- playing Hand on BookshopDoor dialogue
- returning “true”, indicating that this is a scene-specific interaction
|
|
|
Conditions & Scene Variables
There are times in the game when things aren’t always as straightforward as playing a sequential sequence after clicking on something. In these cases, conditional checks are performed based on whether or not certain events have occurred. For example, you cannot board a plane without first presenting your boarding pass to the ticket attendant. It boils down to a simple condition: if you have not presented your boarding pass, the attendant will ask for it. Otherwise, access is granted.
In TSL, this situation is similar to when Graham must first pay Hassan in order to rent his ferry. The checks are performed, as shown:
Written script:
USE gold on Hassan:
A) Before showing Coin to Hassan:
NARRATOR: Graham would be better if this man knew for certain who he is first.
B) After talking to Hassan:
Sequence trigger:
EXT – DOCKS STORM - DAY
GRAHAM: (offering gold) Would this gold be sufficient payment for buying your business.
HASSAN: (eyeing the coins) Hmm… How much is that?
GRAHAM: It should be enough to keep you in business for at least a week. And if it’s not, I’ve got more.
HASSAN: (he takes the gold, he is surprised) in the name of the seven seas this is… (beat) e-hem, as much money as you may think this is, I’ve got my crew to support, myself to support… (pause, almost talking to himself) But on the other hand, if it weren't for your son, I wouldn't have such a thriving business in the first place…
GRAHAM: I have more gold. I’ll pay whatever it takes.
HASSAN: Alright, alright. Your story has touched me. I’ll rent her to you for two days for this quantity, just out of respect for my king. But that’s two days. No more. If the third morning comes and you still need her, I’ll expect a little more than what you’re paying.
GRAHAM: Thank you, captain.
HASSAN: Whenever you are ready.
(End of Sequence)
Game script:
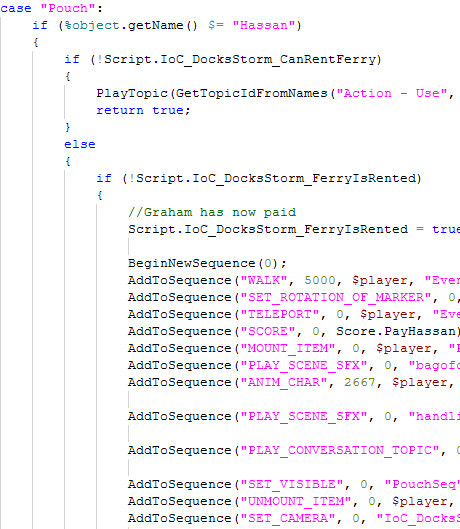
Figure 3: Code for Isle of the Crown - DocksStorm -
Use Pouch on Hassan
You’ll notice that this sequence isn't too different from the Hand on DoorBookShop sequence shown earlier. The main difference is that this sequence is dependent on if conditions and several scene variables. The Script.IoC_DocksStorm_CanRentFerry represents a scene variable that can either be true or false. Keeping this in mind, you can see that this sequence only happens:
- if you click the Pouch on Hassan, i.e.:
if (%object.getName() $= "Hassan")
- if Graham has identified himself so that he can rent the ferry, i.e.:
if (Script.IoC_DocksStorm_CanRentFerry)
- if Graham has not already paid to rent the ferry, i.e.:
if (!Script.IoC_DocksStorm_FerryIsRented)
|
|
|
While there are many more intricacies and special cases to scripting not explained here, the process is not much different from what was described in this entry. The majority of code revolves around creating “sequences”, based on specific user actions and conditions that verify the game’s current state. A sequence is played out upon successful completion of a puzzle, while a dialogue message is played on any erroneous attempt.
Once scene sequences have been scripted, we return to the audio department once again. Voices and music should already be completed at this point, and any re-work performed on those two assets is minimal. The remaining two audio assets will be discussed in next month’s entry. If you haven’t figured out what they are yet, it sounds to me like you need to get out and enjoy the environment.
>> Comments |
|
|
|